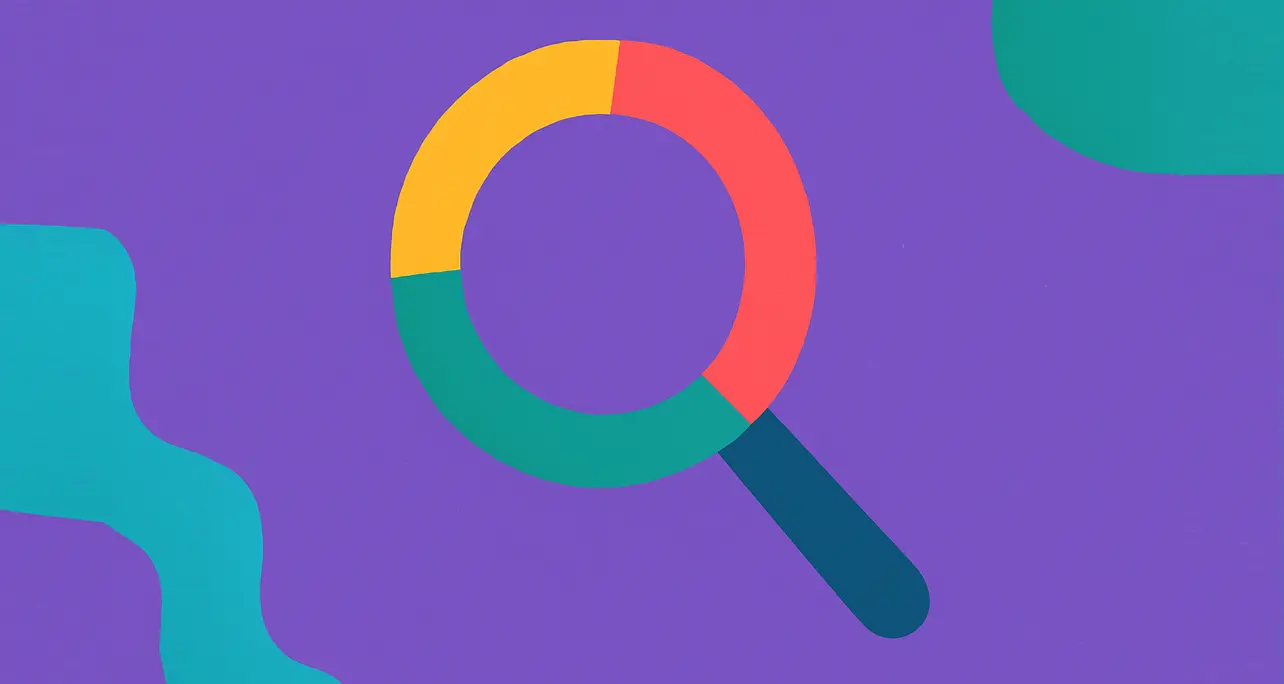
Customizing the WordPress Search Form with Bootstrap 5
WordPress includes a built-in get_search_form()
function to render a search field, but the default version is plain and unstyled. If you’re using Bootstrap 5 or want more design control, here’s how we created a fully customized, responsive search form that fits beautifully into any theme.
🔍 Step 1: Add the Search Form to Any Template
Add this PHP snippet to any part of your theme where you want a search field to appear — in the header, sidebar, or the search results page (like search.php
):
<?php get_search_form(); ?>
This ensures the form is reusable and consistent across the site.
🧰 Step 2: Create a Custom searchform.php
To override the default search form, create a new file in your active theme folder:
/wp-content/themes/your-theme/searchform.php
Then add this custom Bootstrap 5 search form:
<?php
/**
* Custom search form using Bootstrap 5
*/
?>
<form role="search" method="get" class="search-form d-flex w-100 mb-4" action="<?php echo esc_url( home_url( '/' ) ); ?>">
<input
type="search"
class="form-control me-2 search-field"
placeholder="Search…"
value="<?php echo get_search_query(); ?>"
name="s"
aria-label="Search"
/>
<button type="submit" class="btn btn-primary">
<i class="bi bi-search"></i> Search
</button>
</form>
This version uses Bootstrap spacing utilities and includes a search icon from Bootstrap Icons (bi bi-search
). You can replace or remove the icon as needed.
🎨 Step 3: Fix Field Width with CSS
If the field is too narrow or won’t stretch as expected, use the following CSS:
.search-form {
width: 100%;
max-width: 100%;
}
.search-field {
width: 100% !important;
max-width: none !important;
box-sizing: border-box;
}
This ensures both the form and input expand to fill the space in any layout.
✅ Result
- Clean, responsive search field
- Consistent layout using Bootstrap 5
- Works across templates including the search results screen
- Easy to maintain using
get_search_form()
Now you have a fully customized WordPress search experience — modern, mobile-friendly, and styled your way.
About the Author
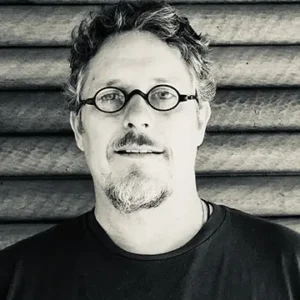
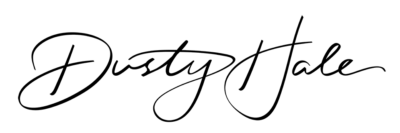
WordPress includes a built-in get_search_form()
function to render a search field, but the default version is plain and unstyled. If you’re using Bootstrap 5 or want more design control, here’s how we created a fully customized, responsive search form that fits beautifully into any theme.
🔍 Step 1: Add the Search Form to Any Template
Add this PHP snippet to any part of your theme where you want a search field to appear — in the header, sidebar, or the search results page (like search.php
):
<?php get_search_form(); ?>
This ensures the form is reusable and consistent across the site.
🧰 Step 2: Create a Custom searchform.php
To override the default search form, create a new file in your active theme folder:
/wp-content/themes/your-theme/searchform.php
Then add this custom Bootstrap 5 search form:
<?php
/**
* Custom search form using Bootstrap 5
*/
?>
<form role="search" method="get" class="search-form d-flex w-100 mb-4" action="<?php echo esc_url( home_url( '/' ) ); ?>">
<input
type="search"
class="form-control me-2 search-field"
placeholder="Search…"
value="<?php echo get_search_query(); ?>"
name="s"
aria-label="Search"
/>
<button type="submit" class="btn btn-primary">
<i class="bi bi-search"></i> Search
</button>
</form>
This version uses Bootstrap spacing utilities and includes a search icon from Bootstrap Icons (bi bi-search
). You can replace or remove the icon as needed.
🎨 Step 3: Fix Field Width with CSS
If the field is too narrow or won’t stretch as expected, use the following CSS:
.search-form {
width: 100%;
max-width: 100%;
}
.search-field {
width: 100% !important;
max-width: none !important;
box-sizing: border-box;
}
This ensures both the form and input expand to fill the space in any layout.
✅ Result
- Clean, responsive search field
- Consistent layout using Bootstrap 5
- Works across templates including the search results screen
- Easy to maintain using
get_search_form()
Now you have a fully customized WordPress search experience — modern, mobile-friendly, and styled your way.
About the Author
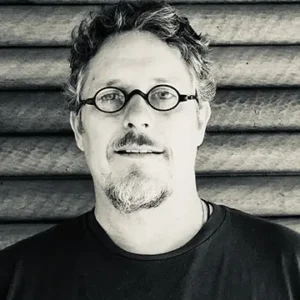
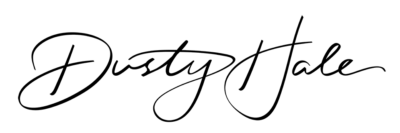